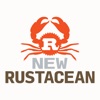
e027: Trust Me; I Promise!
New Rustacean
English - December 01, 2018 04:46 - 21 minutes - 19.7 MB - ★★★★★ - 79 ratingsTechnology News Tech News rust programming programming languages software Homepage Download Apple Podcasts Google Podcasts Overcast Castro Pocket Casts RSS feed
An intro to unsafe Rust and Rust’s idea of safety.
Show Notes
Errata
A quick correction: on the show I said that a trait needed to be unsafe when it had an unsafe fn method. This isn’t correct: safe traits can have unsafe methods, and unsafe traits can exist without any methods at all (as implied by my reference to Send and Sync). You can see this in practice in the following example, which compiles just fine!
trait ASafeTrait {
unsafe fn unsafe_method() {}
}
unsafe AnUnsafeTrait {}
The idea of an unsafe trait is that it has some conditions which you must uphold to safely implement it – again, just as with Send and Sync. In the case of most traits, this will be because some trait method has invariants it needs to hold else it would cause undefined behavior. For another example of this, see the (unstable as of the time of recording) trait std::iter::TrustedLen.
Thanks to Rust language team member @centril for noting this to me after listening when I was recording the show live!
Links
The Rust Programming Language, Chapter 19: Unsafe
The Nomicon
“Rust and OpenGL from Scratch”, by Nerijus Arlauskas
Examples
Borrow-checked code in unsafe
let mut f = String::from("foo");
unsafe {
let borrowed = &mut f;
let borrow_again = &f;
println!("{}", borrowed);
// This would be unsafe and throw an error:
// println!("{}", borrow_again);
}
Safely mutating a raw pointer
let f = Box::new(12);
let mut g = Box::into_raw(f);
g = &mut (g + 10);
Sponsors
Thanks to Parity for sponsoring the show again. Go check out their Rust jobs!
Patreon Sponsors
Adam Green
Aleksey Pirogov
Alexander Payne
Alexander Kryvomaz
Andrew Thompson
Anthony Deschamps
Anthony Scotti
Behnam Esfahbod
Benjamin Wasty
Brandon ‘Spanky’ Mills
Brian Casiello
Brian Manning
Brian McCallister
Bryan Stitt
Bryce Johnston
Caryn Finkelman
Cass Costello
Chap Lovejoy
Charlie Egan
Chip
Chris Palmer
Daniel
Dan Abrams
Daniel Bross
Daniel Collin
Daniel Mason
David Hewson
Derek Morr
Doug Reeves
Eugene Bulkin
Fábio Botelho
Gaveen Prabhasara
Graham Wihlidal
Henri Sivonen
Ian Jones
“Jake”“ferris”" Taylor"
Jako Danar
James Cooper
James Hagans II
Jerome Froelich
John Rudnick
Jon
Jonathan Knapp
Jonathan Turner
Joseph Hain
Joseph Marhee
Justin Ossevoort
Kai Yao
Keith Gray
Kilian Rault
Lee Jenkins
Luca Schmid
Luiz Irber
Lukas Eller
Martin Heuschober
Masashi Fujita
Matt Rudder
Matthew Brenner
Matthias Ruszala
Max Jacobson
Max R.R. Collada
Messense Lv
Micael Bergeron
Michael Mc Donnell
Michael Sanders
Nathan Sculli
Nick Coish
Nick Gideo
Nick Stevens
Nicolas Pochet
Olaf Leidinger
Oliver Uvman
Oluseyi Sonaiya
Ovidiu Curcan
Pascal
Patrick O’Doherty
Paul Naranja
Paul Osborne
Peter Scholtens
Peter Tillemans
Ralph Giles
Ramon Buckland
Randy MacLeod
Raph Levien
Richard Dallaway
Rob Tsuk
Robert Chrzanowski
Ryan Blecher
Ryan Osial
Scott Moeller
Sebastián Ramírez Magrí
Simon Dickson
Simon G
Steffen Loen Sunde
Steve Jenson
Steven Knight
Steven Murawski
Stuart Hinson
Tim Brooks
Tim Süberkrüb
Tom Prince
Toolmaker’s Guild
Ty Overby
Tyler Harper
Victor Kruger
Will Greenberg
William Roe
Zak van der Merwe
Zachary Snyder
Zaki
(Thanks to the couple people donating who opted out of the reward tier, as well. You know who you are!)
Become a sponsor
Patreon
Venmo
Dwolla
Cash.me
Flattr
PayPal.me
Contact
New Rustacean: + Twitter: @newrustacean + Email: [email protected]
Chris Krycho + GitHub: chriskrycho + Twitter: @chriskrycho