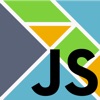
59: Getting Haskell Unit 1 (part 2)
Javascript to Elm
English - November 22, 2018 04:02 - 26 minutes - 22.5 MB - ★★★★★ - 4 ratingsTechnology elm javascript programming Homepage Download Apple Podcasts Google Podcasts Overcast Castro Pocket Casts RSS feed
We are cruising right along. Getting some of the finer details of list, recursion, closure, and pattern matching in Haskell down!
Corrections and feedback WOW, didn’t even mention immutability last week. When? at any point? Ever! Several of the examples we went over would have benefited from a mention that variables in Haskell are IMMUTABLE I put it in bold to make up for the lack of it last week. Maybe? Right? Also missed spoke about partial application. I said once the function gets all of it’s arguments, then and only then will it evaluate and return. But in Haskell that is not the case. If fact, it’s the exact opposite! Haskell is lazily evaluated so until that return value is needed, Haskell won’t do anything with it. Say like an infinity list, that is totally ok in Haskell, it’s not going to cause a stack overflow, or throw an exception. —we’ll get more into that later. Lists
This is a big one. So much of the data structure and transforming is using lists! So we’ve got to get a really good understanding of this if we want to move forward and not fall on our faces. And by faces, I mean get over that hump and really understand Haskell and the great things it has to offer.
Side rant. Damn it there are a lot of updates. I mean like a lot a lot
Starting simple. Head and Tail
Prelude> head [1, 2, 3] 1 Prelude> head [] *** Exception: Prelude.head: empty list Prelude> You’ll notice that head of an empty list returns an Exception! and they do not return a Maybe Type like they do in Elm.The cons operator. This eluded me for such a long time. I kept getting it confused with a punctuation for a type signature. And frankly never stopped to understand the basic syntax of Haskell. Sure, I read some of the documentation. But I didn’t explicitly type it out like I am not, and it constantly came back to bit me in the ass. So what is cons ? it’s an infix operator, (cons is short for construct. like construct a list. get it?) So we take a value and “cons” it to a list. And we have a list. And a list can always be represented by a value ‘consed’ with a list.
Every element of a list MUST be the same type. Whether that’s string, char, tuples, whatever.
I like the examples that ‘desugar’ some of the syntax in steps.
Like double quotes is really a list of of single quote letters or charsThen we can concat with ’++’
Common List functions
!! operator : takes a list and number and returns the element at that index of the List length : takes a list reverse: um, reversed the order of the list silly.elem: takes a value and a list and returns True/False if it’s in that List
List Final
Write a function subseq that takes 3 arguments. A start, an end, and a list and returns the subsequence between the start and end Prelude> subseq start end list = take diff list where diff = end - start Prelude| Prelude> subseq 1 5 [1..10] [1,2,3,4] Prelude> subseq 3 5 [1..10] [1,2] Prelude>ok missed the drop part, and that the start and end ARE the indexes making this WAY easier
Prelude> subseq start end xs = take (end - start + 1) (drop start xs) Prelude> subseq 2 5 [1..10] [3,4,5,6] Prelude> subseq 3 5 [1..10] [4,5,6] Prelude> subseq 3 5 [10..20] [13,14,15] Prelude> subseq 2 7 "a puppy" "puppy" Prelude> drop 2 "a puppy" "puppy" Prelude> take 6 "puppy" Recursion, Pattern MatchingAs we just learned. We don’t have looping functions like for, while, and until. Which is instinctively where my brain goes. When presented with a list.
HOC No, not Higher Order Components.
Higher Order Functions!
Functional OOP wha? Resources Get Programming With Haskell FollowJavaScript to Elm
Twitter: @jstoelmEmail: [email protected]
Jesse Tomchak
Twitter: @jtomchak